Display your continuous integration build status on Jira
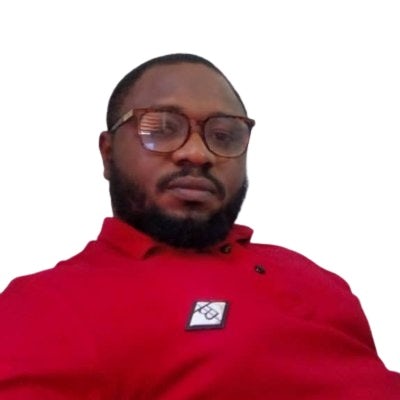
Fullstack Developer and Tech Author
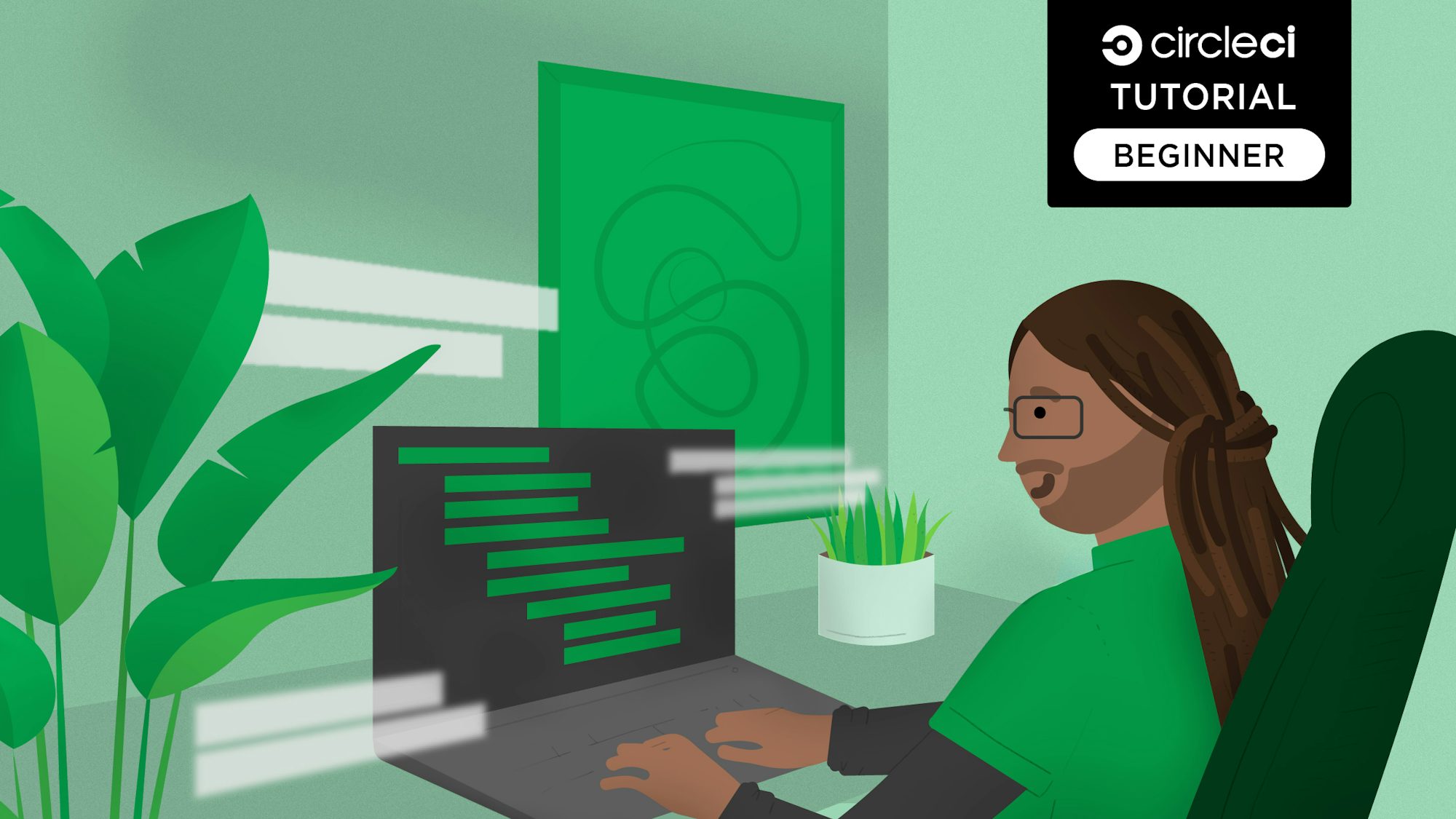
Automating tests and deployments with CI/CD makes development more productive. Instead of managing multiple tools and manual processes, all they have to do is commit the code to a code repository.
Not everyone on a project manages the CI/CD system, but they may need to know when the build process fails or is successful. This is where a proper handshake between CI/CD systems and project management tools such as Jira shines.
Jira is well known and used by many, if not most, software development teams as a project management software for tracking issues, managing Scrum and Agile projects, and more.
In this tutorial, I will show you how to set up integration between your CI/CD workflows (CircleCI for this project) and Jira work items. With this implementation, you have access to the status of all your builds and deployments right within your Jira panel. To make this integration happen, you will use the Jira orb from the CircleCI orb registry.
Prerequisites
For this tutorial, you will need:
- Node.js installed on your computer
- A GitHub account
- A CircleCI account
- A Twilio account
- An Atlassian account
- Admin permissions on JIRA
What we will build
To monitor the status of your workflow, you will build a basic Node.js application that returns a message from the homepage route /
. You will also write a test assert that the expected message was returned.
Getting started
Create a folder for the project and move into the newly created folder using this command:
mkdir node-jira-app && cd node-jira-app
Next, create a package.json
file with dynamically defined values within the project:
npm init -y
Installing project dependencies
To build the application, we will use Express as the Node.js express web application framework. Run this command to install Express:
npm install express --save
We will use Mocha to run tests for the project and Chai as the asserting library. Issue this command to install both libraries:
npm install chai mocha request --save-dev
Once the installation process is completed, create a new file named index.js
within the root of the project:
touch index.js
Open the new file and populate it with this content:
var express = require("express");
var app = express();
// Root URL (/)
app.get("/", function (req, res) {
res.send("Sample Jira App");
});
//server listening on port 8080
app.listen(8080, function () {
console.log("Listening on port 8080!");
});
The content you added specifies the message that should be displayed at the homepage of the project.
Next, run the application with:
node index.js
Navigate to http://localhost:8080
from your browser.
The result is just a basic message to confirm that our application works. Stop the application from running by typing CTRL + C
in your terminal. Press Enter to proceed.
Create a test script
To get started, create a separate folder within the application to house the test scripts. Name it test
.
mkdir test
Add a new file to the test
folder and name it test-home.js
:
touch test/test-home.js
Next we will add a simple test to confirm the home page contains the text “Sample Jira App”. Paste this content into the file:
var expect = require("chai").expect;
var request = require("request");
it("Home page content", function (done) {
request("http://localhost:8080", function (error, response, body) {
expect(body).to.equal("Sample Jira App");
done();
});
});
Run the test locally
First, update the scripts
section in the package.json
file with a command that runs both the application and test script easily. Enter this content:
{
"name": "jira-app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "mocha",
"start": "node index.js"
},
...
}
}
Run the application with npm run start
. From a different tab but still within the project, issue this command to run the test:
npm run test
This will be the result:
➜ node-jira-app npm run test
> jira-app@1.0.0 test
> mocha
✔ Main page content
1 passing (34ms)
Adding CircleCI configuration to automate the test
To automate the test, create a .circleci
folder inside the root directory of your application. Add a new file named config.yml
to it. Open the newly created file and paste this code:
version: 2.1
orbs:
node: circleci/node@4.7.0
jobs:
build-and-test:
executor:
name: node/default
steps:
- checkout
- node/install-packages
- run:
name: Run the app
command: npm run start
background: true
- run:
name: Run test
command: npm run test
workflows:
build-and-test:
jobs:
- build-and-test
This configuration file specifies which version of CircleCI to use. It uses the CircleCI Node orb to set up and install Node.js. The config also installs all the dependencies for the project.
The final command is the actual test command, which runs our test.
Before you deploy the project to GitHub, create a .gitignore
file in the root directory of your project. Enter this:
# compiled output
/dist
/node_modules
# Logs
logs
*.log
npm-debug.log*
yarn-debug.log*
yarn-error.log*
lerna-debug.log*
# OS
.DS_Store
# IDEs and editors
/.idea
.project
.classpath
.c9/
*.launch
.settings/
*.sublime-workspace
# IDE - VSCode
.vscode/*
!.vscode/settings.json
!.vscode/tasks.json
!.vscode/launch.json
!.vscode/extensions.json
Now you can push the project to GitHub.
Connecting the application to CircleCI
At this point, you need to connect the application to CircleCI. To do that, log in to your CircleCI account with the linked GitHub account that contains this application. Go to the Projects page on the CircleCI dashboard. Select your GitHub account and add the project.
Click Set Up Project. You will be prompted to either create a new config.yml
file or use an existing one by selecting the branch you pushed your project to.
Click the Let’s Go button and CircleCI will start running the project.
Your build should be a success.
Connecting Jira with CircleCI
Enabling successful interaction between CircleCI and Jira software requires few steps. Here they are in order:
- Create a project and Issue on Jira.
- Install CircleCI for Jira plugins.
- Add Jira token to CircleCI.
- Create a Personal API token on CircleCI.
- Use CircleCI version 2.1 at the top of your
.circleci/config.yml
file. - Add the orbs stanza below your version, invoking the Jira orb.
- Use
jira/notify
command in your existing jobs to send status to the Jira development panel.
Create a Jira project and issue
Log in to your Jira instance here and create a project. On the project page, click the Create button.
Give it a name that best describes the issue. For the tutorial, we used Build Status Demo App Issue
.
When the issue is created, you can review it in detail by clicking on the issue in the list.
Be mindful of the key for this issue; it will be used to uniquely identify the issue in CircleCI.
Install CircleCI Jira plugins
Navigate to your Jira dashboard and explore the Marketplace. Search for the CircleCI for Jira app.
Note: You must be a Jira admin to install the plugins.
Click, then follow the instructions to install the plugins and set them up.
Click the Get Started button to get your Jira configuration token.
Copy this token and keep it somewhere safe. You will need it later in the tutorial. This token ensures and enforces proper connection between your Jira instance and CircleCI projects.
Add the Jira token to CircleCI
From the application’s pipeline page on CircleCI, click the Project Settings button.
You will be redirected to a page where you can select Jira Integration from the sidebar. Click the Add Token button, which is in the Set up Jira authentication section. This will show a prompt where you can enter the Jira configuration token.
Save and add the token once you are done.
Create a Personal API Token
Go to User Settings and click the Create New Token button.
Give your token a friendly name.
Click Add when you are done. Copy the generated token and go back to the Project Settings page.
From there click Environment Variables from the sidebar, and then click Add Environment Variable. Name the variable CIRCLE_TOKEN
and use the generated API token as its value.
You have successfully connected Jira instance and CircleCI pipeline.
Enabling CircleCI for Jira software
Before you can start showing the status of your builds from CircleCI on Jira, you need to make some modifications to the CircleCI configuration file.
Back in your project, open .circleci/config.yml
and update its content by adding the Jira orb:
version: 2.1
orbs:
node: circleci/node@4.7.0
jira: circleci/jira@1.3.1
jobs:
build-and-test:
executor:
name: node/default
steps:
- checkout
- node/install-packages
- run:
name: Run the app
command: npm run start
background: true
- run:
name: Run test
command: npm run test
workflows:
build-and-test:
jobs:
- build-and-test:
post-steps:
- jira/notify
We included a Jira orb and the workflow as well. The jira/notify
command will be used to send the status of builds to the Jira instance.
Update Git and push the project back to GitHub. This time, create a features branch using the key for the task or issues id on Jira. In our case it is BSDP-1
.
git checkout -b features/BSDP-1
Do not forget to replace BSDP-1
with your key if it is different.
Conclusion
Among other benefits, connecting CircleCI to Jira software keeps everyone in your development team up-to-date with the status of every build without needing to go to the pipeline dashboard on CircleCI.
In this tutorial, you:
- Built a simple Node.js application
- Wrote a test script for it
- Followed the steps to connect a CircleCI pipeline with a Jira item
- Displayed the status of the CircleCI builds on Jira
I hope you found this tutorial helpful. If you did, share it with your team!