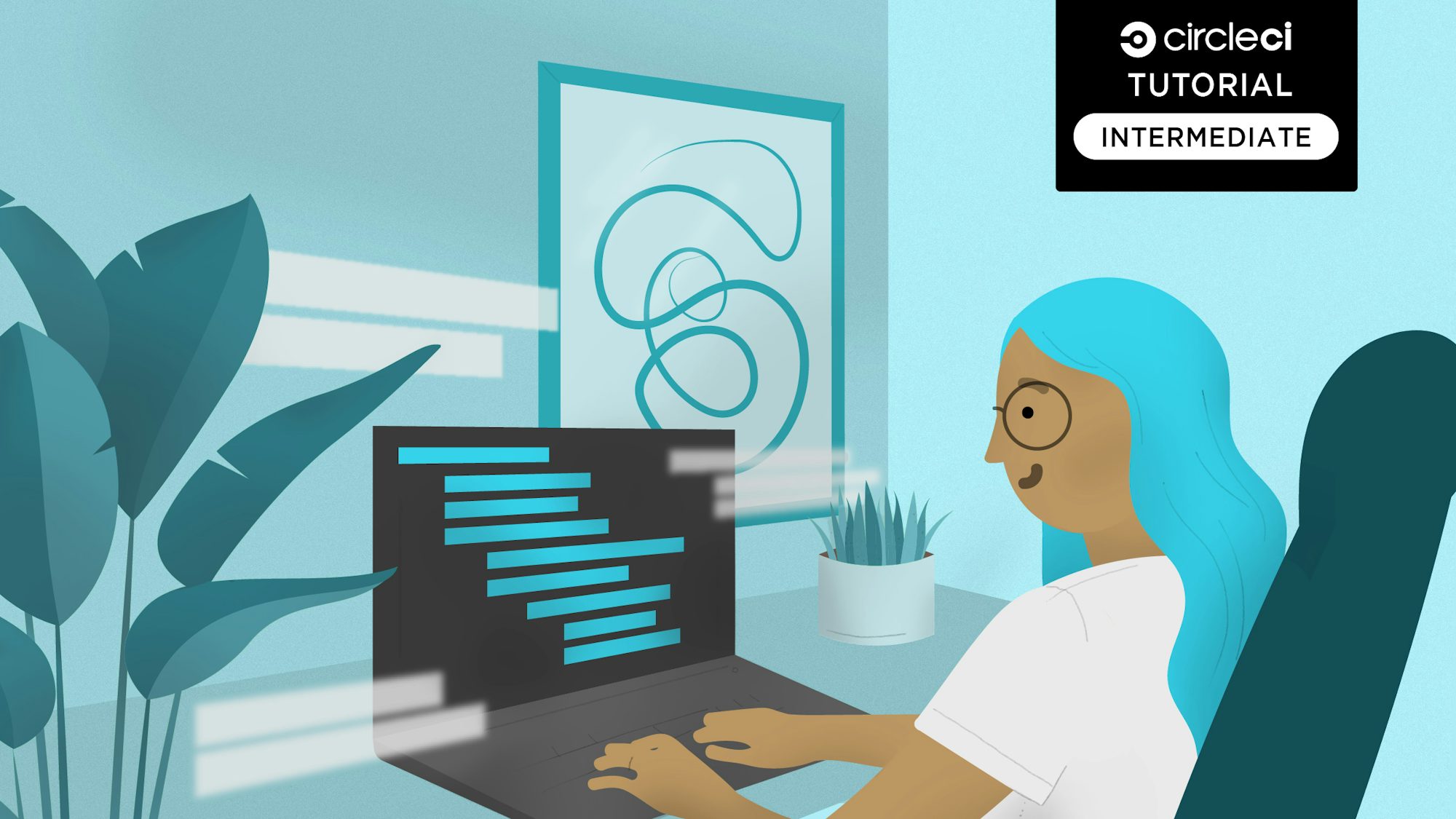
Ensuring that an application renders properly in any browser size is important for user experience but tricky to get right. With users accessing your application on devices that have a wide range of screen sizes and aspect ratios, it’s difficult to guarantee that your application will look and function as intended for every user.
Viewport testing ensures that web applications work as intended in different device screen sizes. In this tutorial, you will learn the importance of using Cypress to test viewports for browser and mobile versions of an application. You’ll learn how to add viewport tests to an application, and then you’ll integrate CircleCI as part of the CI/CD process to automate your viewport tests, giving you continuous feedback on your application’s responsiveness and functionality across various devices.
Prerequisites
To follow along with this tutorial, you will need the following:
- A GitHub account account
- A CircleCI account
- NodeJS installed locally
- Cypress V9 installed
- A basic understanding of JavaScript and Cypress
Setting up the sample application
To simplify [blank] there is a simple application that you can use for this tutorial. Follow these steps to clone and try out the application.
First, clone the application from the GitHub repository:
git clone https://github.com/CIRCLECI-GWP/viewport-testing-with-cypress.git
Then, cd
into the cloned directory:
cd viewport-testing-with-cypress
Next, install the dependencies needed for this tutorial. Execute this command:
npm install
Finally, run npm start
and visit the application in your browser at localhost:9000
.
What are viewports?
A viewport is the area of a webpage that a user can see on their display. This varies depending on the type of device or display, as well as the window on which a webpage is being displayed.
Most web traffic is generated through mobile devices, so most web applications are optimizing interfaces for these devices. Testing viewports ensures that no matter what device or screen size is in use, the functionality is similar if not better while still ensuring that the performance is optimal.
Here’s an illustration of the different steps a user might take when using the browser and mobile viewports:
We are using Cypress to test viewports because you can write tests faster and have an overview of the different test cases, while at the same time using its ability to run on the browser.
Cypress also allows you to control the dimensions of the viewport and therefore test for multiple viewports at once. It is important to know that for Cypress to achieve this, it just sets the browser’s width and height to the device resolution that is under test.
Cypress configuration
Open a new terminal while in the viewport-testing-with-cypress
directory. It is important that you keep open the terminal window that is running your application from the npm start
command.
In your new window, start Cypress. Run:
npx cypress open
Cypress will open the dashboard, where you can choose which tests to run. Select Run 1 integration spec to run all the integration tests (in this case, there is only one). Cypress will launch a Chrome window with the test output.
The first two two test cases ensure the webpage loads without issues; these tests pass successfully. The tests live in travel.test.js
in the integration folder of the Cypress folder for this tutorial. They look like this:
/// <reference types="cypress" />
describe("Cypress Viewport Tests Suite", () => {
it("should be able to load the page", () => {
cy.visit("http://localhost:9000")
})
it("should load the features page", () => {
cy.get("a[id=featuresBtn]").click()
cy.contains("Your favorite places are waiting! Let us help bring your dreams to life")
})
})
In these two tests, Cypress visits the site’s URL to ensure that it loads properly without errors. Cypress clicks the features button and checks whether the resulting page contains “Your favorite places are waiting! Let us help bring your dreams to life”.
The rest of the tests are broken into two groups: those for the desktop viewport and those for the mobile viewport.
Cypress uses the Mocha
framework and Chai
assertion library to help with the organization of tests. One of the extended features is context()
, which the example uses to differentiate the viewports for different devices.
The next code snippet lives in the test suite of the travel.test.js
file, and handles the desktop viewport tests.
context("desktop viewport", () => {
beforeEach(() => {
cy.viewport(1536, 960)
// Runs the tests in this context for the resolution defined
})
it("displays the whole navigation bar", () => {
cy.get(".navbar").should("be.visible")
cy.get("div[id=menu-btn]").should("not.be.visible")
})
})
This is the test for the desktop resolution, where you ensure that the entire navigation bar is displayed. It uses the cy.get() command to access the elements and selectors. It then uses the should() assertion to ensure that the entire navigation bar is visible. Because all the links to pages can fit within this large viewport, the next line ensures that we don’t show the hamburger menu.
The next test verifies mobile. For this test makes sure that the toggle button is visible and you can access link elements to other pages by clicking it.
context("mobile viewport", () => {
beforeEach(() => {
cy.viewport("iphone-6")
// Runs the tests in this context for the resolution defined
})
it("displays the hamburger menu", () => {
cy.get("div[id=menu-btn]").should("be.visible")
})
it("clicks the toggle button", () => {
cy.get("div[id=menu-btn]").should("be.visible").click()
cy.get(".content").should("be.visible")
})
}
)
This code snippet shows the different ways to pass a viewport configuration to your Cypress tests. We can do it either in terms of the screen resolution or common mobile device names like the iphone-6
. For more information on the arguments to pass to the method, you can check the Cypress documentation on viewport arguments.
Configuring CircleCI
CircleCI is a CI/CD platform that automates test runs. The project contains a .circleci
folder in the root directory with a config.yml
file where you have our configuration. This project’s config.yml
is straightforward:
version: 2.1
orbs:
cypress: cypress-io/cypress@1
workflows:
build:
jobs:
- cypress/run
You are using CircleCI’s Cypress orb, which is a pre-built configuration that installs and configures dependencies, caches commands on CircleCi, and does all the heavy lifting for your configuration.
To run the test for this project in your own CircleCI account, push your changes to a new remote repository on GitHub. You can do this by creating an empty repository on GitHub and then running this in the root of the example project:
git remote set-url <URL-OF-YOUR-NEW-REPO>
Next, push the example project to your GitHub with:
git push
Log into your CircleCI account. On the Projects tab, click Create Project. Select Github and choose the repository from the dropdown.
Click Create Project. CircleCI detects the config.yml
file in the GitHub repository. You’ll be taken to your project page, where you’ll see that there have been no runs of your pipeline yet. Push a small commit that doesn’t functionally change anything, like changing the name of a test, to trigger your first pipeline run:
Voila! Just like that, you have set up your project and integrated CircleCI into it. Now, any time you update your project code, CircleCI will automatically trigger your Cypress tests, giving you the feedback you need to move quickly and confidently.
Conclusion
In this tutorial, you’ve learned how to use Cypress to test viewports. You then used the Cypress orb to integrate CircleCI into your development cycle and automate your test runs.
Testing viewports is important to ensure a great user experience no matter the screen sizes or orientation of the contents of a web application. I hope you enjoyed reading this tutorial as much as I did creating it. Until the next one, keep learning!