Automate deployment of React applications to Firebase
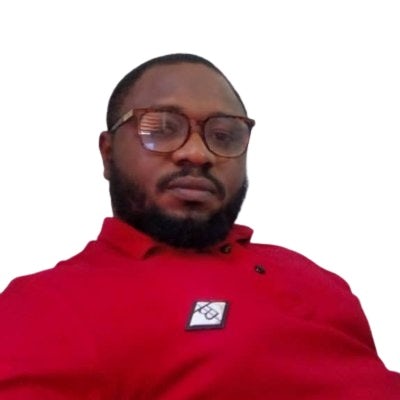
Fullstack Developer and Tech Author
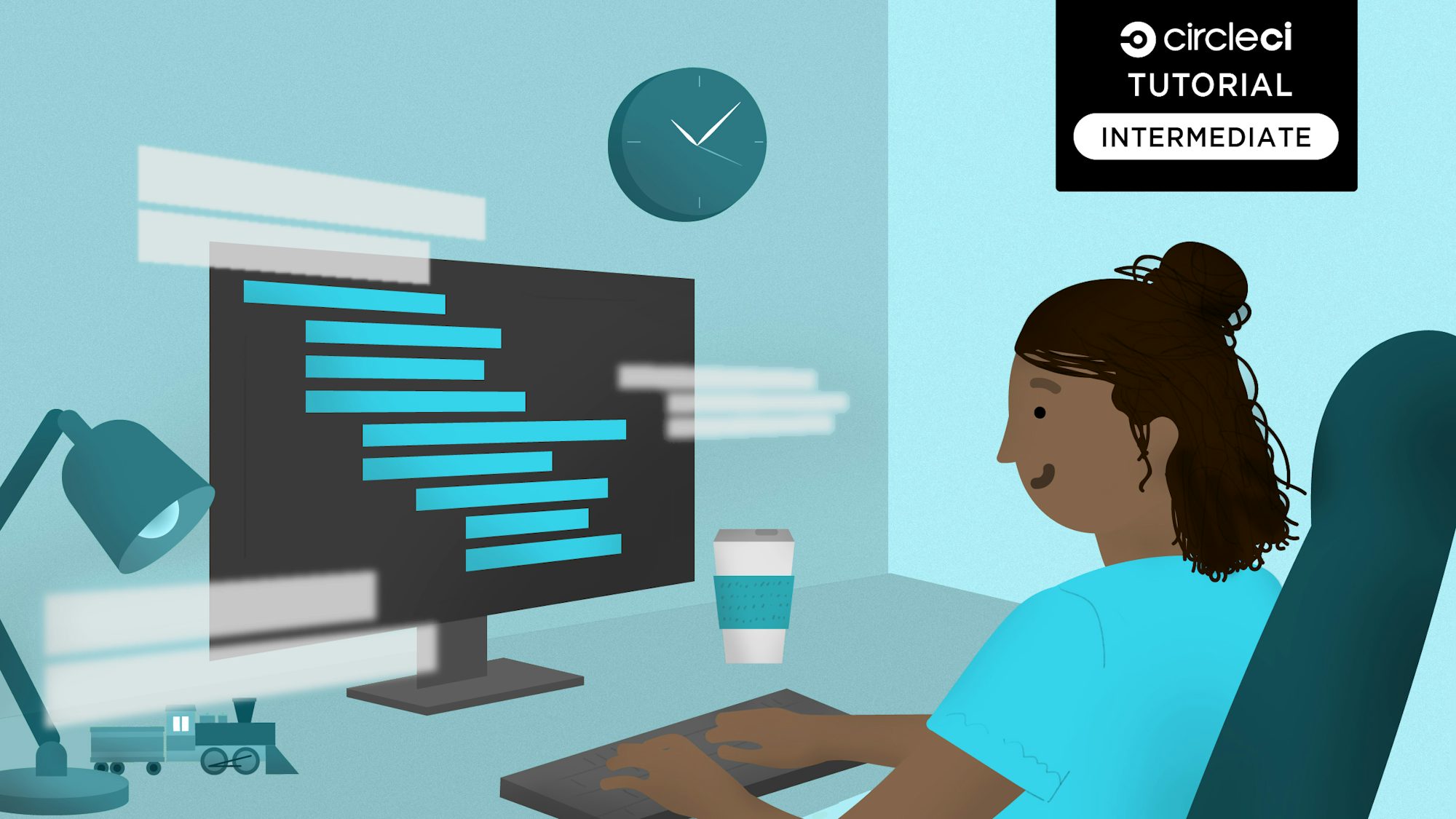
Many platforms offer free hosting services for React and other JavaScript frameworks. These frameworks can be used for building single-page applications, which is handy when you need to launch a minimum viable product or a quick proof of concept.
Firebase is a development platform developed by Google that provides file storage, hosting, database, authentication, and analytics. Firebase is free, provides an SSL certificate by default, and offers impressive speed across multiple regions. Your fellow developers are taking advantage of these tools, and you can too.
In this tutorial, you will learn how to host your React application on Firebase by setting up a continuous deployment pipeline with CircleCI.
Prerequisites
For this tutorial, you will need:
- Node version
>=16.19.0
( LTS/Gallium ). Firebase CLI requires version^14.18.0 || >=16.4.0
. - A CircleCI account.
- A GitHub account.
- A Firebase account.
- Familiarity with building web applications using React.
Our tutorials are platform-agnostic, but use CircleCI as an example. If you don’t have a CircleCI account, sign up for a free one here.
Cloning the demo project
To begin, clone the demo project for this tutorial on GitHub. Use Git to issue the following command:
git clone --single-branch --branch starter https://github.com/CIRCLECI-GWP/react-firebase-app.git
This demo application retrieves the list of dummy users from a free Rest API for testing.
Next, move into the newly cloned app, install its dependencies, and run the application with:
// change directory
cd react-firebase-app
// install dependencies
npm install
// Run the application
npm run start
Go to http://localhost:3000
to review the home page locally.
Now that you know the demo application is working, you are ready to set up a project on Firebase.
Setting up a project on Firebase
You need a free account to access all the features that Firebase has got to offer, so open up a Firebase account and go to the Firebase console page.
Next, use the following steps:
- Click the Add project button.
- Enter a name for your project. I have named mine
react-firebase-app
. Keep in mind that project IDs are unique in Firebase. - Click Continue.
- Disable Google Analytics; it is not required for this project.
- Click Create project again.
You have successfully created a project on Firebase.
Configuring Firebase hosting
To successfully host your application on Firebase, you need to install its tools and initialize it within your project.
Open a new terminal. Run this command to install Firebase tools globally:
npm install -g firebase-tools
Once the installation is completed, you now have global access to Firebase Command Line Interface tools. You can use them to deploy code and assets to your newly created Firebase project.
Connecting React to Firebase
From the terminal, sign in to your Firebase account:
firebase login
This command will open a browser and prompt you to select an account.
Next, ensure that you are within the root of the react-firebase-app
project and issue this command to initialize it:
firebase init
You will be prompted to respond to some questions.
- Choose hosting: Configure files for Firebase hosting and (optionally) set up GitHub Action deploys.
- Use an existing project: Select the Firebase project you created earlier (
react-firebase-app
). - Enter
build
as the public directory. - Configure as a single-page app:
Yes
. - Set up automatic builds and deploys with GitHub:
No
. For this tutorial, we are using CircleCI to run tests and handle deployment.
Here is the output from the terminal:
######## #### ######## ######## ######## ### ###### ########
## ## ## ## ## ## ## ## ## ## ##
###### ## ######## ###### ######## ######### ###### ######
## ## ## ## ## ## ## ## ## ## ##
## #### ## ## ######## ######## ## ## ###### ########
You're about to initialize a Firebase project in this directory:
/Users/yemiwebby/tutorial/circleci/react-firebase-app
? Which Firebase features do you want to set up for this directory? Press Space to select features, then Enter to confirm your choices. Hosting: Configure files for
Firebase Hosting and (optionally) set up GitHub Action deploys
=== Project Setup
First, let's associate this project directory with a Firebase project.
You can create multiple project aliases by running firebase use --add,
but for now we'll just set up a default project.
? Please select an option: Use an existing project
? Select a default Firebase project for this directory: react-firebase-app-7c641 (react-firebase-app)
i Using project react-firebase-app-7c641 (react-firebase-app)
=== Hosting Setup
Your public directory is the folder (relative to your project directory) that
will contain Hosting assets to be uploaded with firebase deploy. If you
have a build process for your assets, use your build's output directory.
? What do you want to use as your public directory? build
? Configure as a single-page app (rewrite all urls to /index.html)? Yes
? Set up automatic builds and deploys with GitHub? No
✔ Wrote build/index.html
i Writing configuration info to firebase.json...
i Writing project information to .firebaserc...
✔ Firebase initialization complete!
The project’s initialization process also generated two unique files at the root of your project. These files are required for successful deployment and must be checked into source control.
firebase.json
contains your project’s hosting configuration. It instructs Firebase CLI about the files in your project directory to upload and deploy..firebaserc
specifies the project to connect to the uploaded code once you successfully deploy to Firebase.
Configuring CircleCI
To configure the deployment pipeline, create a new folder named .circleci
at the root of the project and within the new folder, create a config.yml
file. Open the new file and use the following content for it:
version: 2.1
jobs:
build:
working_directory: ~/project
docker:
- image: cimg/node:18.16.0
steps:
- checkout
- run:
name: Update NPM
command: "sudo npm install -g npm"
- restore_cache:
key: dependency-cache-{{ checksum "package-lock.json" }}
- run:
name: Install Dependencies
command: npm install
- run: npm install --save-dev firebase-tools
- save_cache:
key: dependency-cache-{{ checksum "package-lock.json" }}
paths:
- ./node_modules
- run:
name: Build application for production
command: npm run build
- run:
name: Deploy app to Firebase
command: ./node_modules/.bin/firebase deploy --token=$FIREBASE_TOKEN
workflows:
build-and-deploy:
jobs:
- build
This configuration specifies all the required tools to install and build the React application on CircleCI. It installed the Firebase tool with npm install --save-dev firebase-tools
and set up a command to automatically deploy your application to Firebase once the tests are successful.
The deployment will require the FIREBASE_TOKEN
. You are logged in from the terminal, so you can easily create a token using the Firebase CLI. Enter this command:
firebase login:ci
This opens a browser where you can authenticate your account. The token is then printed in your terminal.
Copy this and save it somewhere convenient. You will need it later on in this tutorial.
The next step is to set up a repository on GitHub and link the project to CircleCI. Review Pushing a project to GitHub for instructions.
Note: GitLab users can also follow this tutorial by pushing the project to GitLab and setting up a CI/CD pipeline for their GitLab repo.
Once your GitHub repository has been updated with the changes, log into your CircleCI account. If you signed up with your GitHub account, all your repositories will be available on your project’s dashboard.
Next, locate your react-firebase-app
project, and click Set Up Project.
You will be prompted to either write a new configuration file or use the existing one. Select the existing one and enter the name of the branch where your code is housed on GitHub. Click Set Up Project.
Your first workflow will start running. The build will fail, though, because you have not yet created the FIREBASE_TOKEN
environment variable.
To fix that, you will need to add FIREBASE_TOKEN
as an environment variable. Click Project Settings and then click Environment Variables on the left sidebar. Create this variable:
FIREBASE_TOKEN
is the value of the token you generated from the terminal earlier.
Back on the dashboard, click Rerun Workflow from Failed. This will trigger the workflow, which now builds successfully.
Go to the hosting URL shown in the previous step. For me the URL is: https://react-firebase-app-7c641.web.app
.
Conclusion
In this tutorial, we reviewed the step-by-step process for hosting a React application on Firebase. You downloaded an existing React application and leveraged CircleCI infrastructure to ensure that the application is deployed without any human interaction.
The processes explained here apply to both existing and new React applications. The complete source code can be found here on GitHub.