Testing an API with Postman
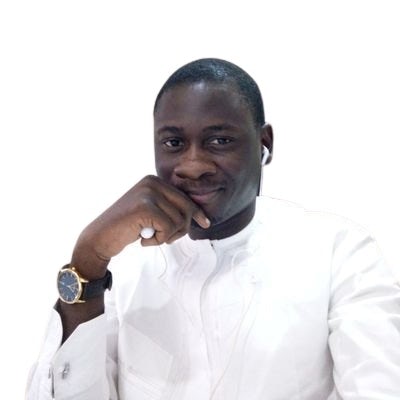
Fullstack Developer and Tech Author
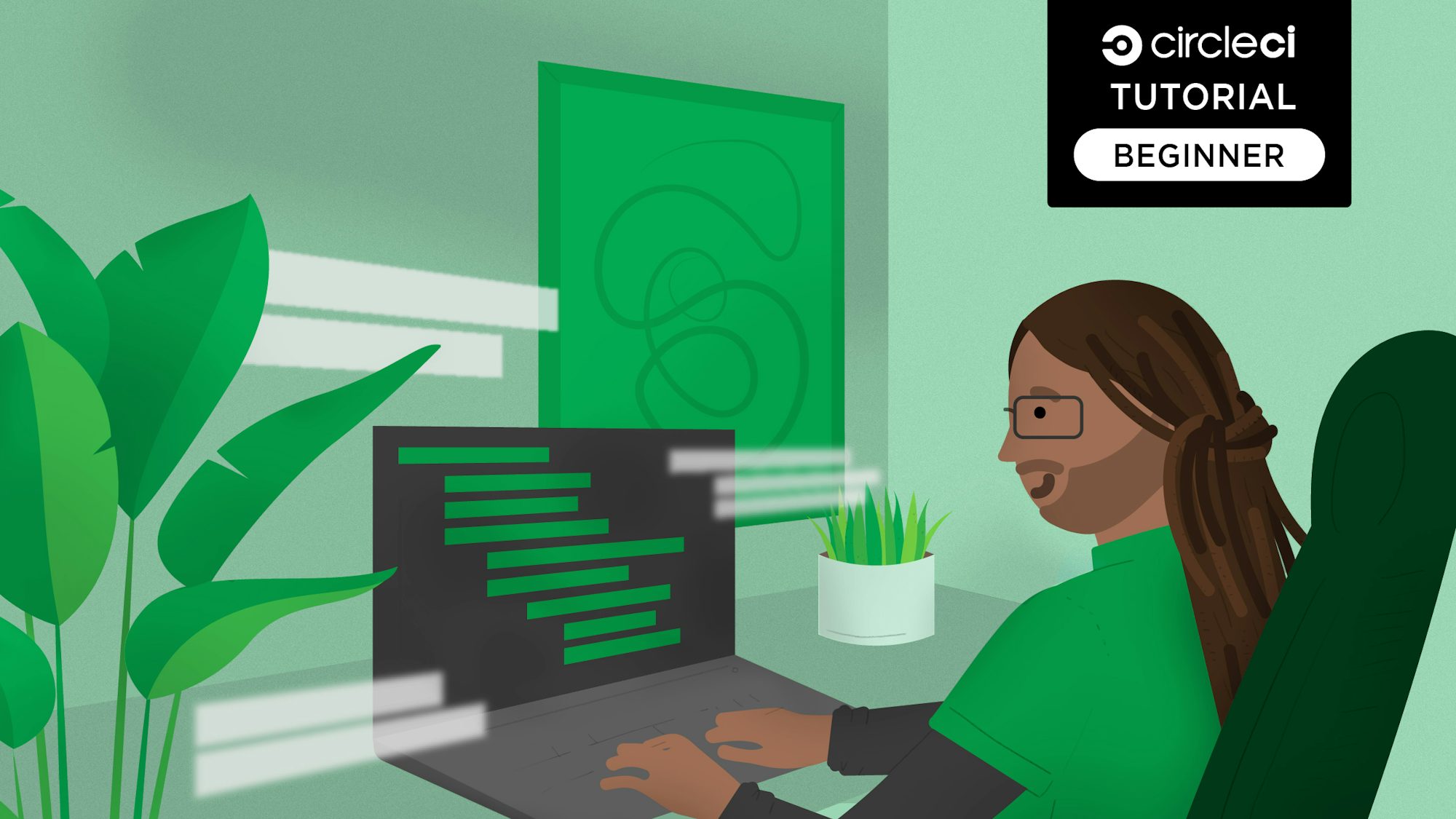
The process of testing APIs has come a long way from the time when cURL was the only tool available. Postman improved the end-to-end testing experience by allowing developers to easily make requests from a user-friendly interface. You can even use Postman as a full-featured collaboration platform for API development and testing.
In this tutorial, you will learn how to perform and automate tests against your APIs using Postman’s command-line utility, Newman.
Prerequisites
To complete this tutorial, a few things are required:
- Basic knowledge of JavaScript
- Postman for Desktop installed on your system. You can download it here. This tutorial uses Version 11.2.0.
- A CircleCI account
- A GitHub account
You will be testing the functionality of a public sample API It’s a simple API that consists of many endpoints, but you’ll only use a few for creating and fetching user accounts.
With everything installed and set up, you can start the tutorial.
Setting up a Postman environment
To set up an automated testing pipeline for your API tests, you will need to create an environment in Postman. Setting up the scope of the environment will help you avoid variables clashing globally or with other environments.
Open Postman desktop and select Environments from the left menu bar. Your environments will be displayed here if you have any. Otherwise, you will have a link to create a new one. Click Create Environment or use the plus icon.
Note: Your Postman UI may look slightly different than the screenshots in this tutorial, but the tools you need will still be available.
Next, enter a name for the environment. Enter the environment variable, api_url
. Fill in the API base URL with https://reqres.in/api
(without a trailing slash). The value in INITIAL VALUE
is duplicated in CURRENT VALUE
. Keep them identical for this tutorial.
Click Save to add the new environment. Select the new environment from the dropdown at the top right of the interface.
Creating a Postman collection for API testing
Your next step is to create a Postman collection for the user endpoints of the API you are testing. Postman collections serve as containers for API requests. They are normally used to group requests relating to a particular entity.
To create a new collection, click the Collections tab on the left sidebar of the interface. Then click Create Collection or the plus icon.
Fill in the name (Users
) of your collection. You can also add a description for the collection to provide more insight. Your new collection is listed on the left sidebar under the Collections tab.
Adding requests to the collection
Now you can add requests to your collection. The sample API consists of two endpoints:
/users
(GET): Fetches a list of user profiles/users
(POST): Creates a new user profile
To add a new request, click the menu icon beside the collection.
Click Add request. Create a request for the GET https://reqres.in/api/users
endpoint, and click Save. Users
is the collection you created earlier.
A new request tab is listed. Enter the endpoint for the request in the address bar ({{api_url}}/users
) using the api_url
variable you created for the current environment. Name it Fetch the list of users
.
Postman displays the returned collection of users with some metadata. Click Save.
Create another request, this time for the POST
{{api_url}}/users
endpoint. This is a POST
request and requires a name
, job
.
Make sure that you save this request.
Writing tests for API requests
Now that set up is complete, it is time to add some tests.
The left sidebar should show that the GET
{{api_url}}/users
request is selected. If it is not, click to select it. Click the Tests (sometimes labeled Scripts) tab and enter this as a Post-res script:
pm.test("Request is successful with a status code of 200", function () {
pm.response.to.have.status(200);
});
pm.test("Check that it returns an array for data", function () {
var jsonData = pm.response.json();
pm.expect(jsonData["data"]).to.be.an("array");
});
The Postman tests you just added are Chai assertions.
In the code above, the first test checks that the request completes with a success status code of 200
. The second test checks to see that the data returned from the request is contains an attribute data
with an array value; in this case, the expected array of user profiles.
Save and run the request again. Details about the tests that have passed are listed on the Test Results tab.
Next, add tests for the POST
request to the POST
{{api_url}}/users
endpoint. Add the following to the Scripts
window for this request:
pm.test("User creation was successful", function () {
pm.expect(pm.response.code).to.be.oneOf([200, 201, 202]);
});
pm.test("Confirm job was assigned correctly", function () {
var jsonData = pm.response.json();
pm.expect(jsonData["job"]).to.eql("writer");
});
This code’s first test asserts a successful response by checking the status codes 200
, 201
, and 202
. These codes are used to indicate a successful POST
request. The second test checks the job
of the returned json
object to make sure it matches the provided job
.
Run the requests and the tests will pass.
Setting up a test automation project
So far you have used the Postman GUI to make requests. That’s great, but the goal of this tutorial is automating testing and generating test results. That makes your next task setting up a project for test automation.
To get started, create a folder for the project at any location on your local machine:
mkdir postman-api-testing
Make sure you have saved your requests. Then click Export from the Collection Context menu.
A file named Users.postman_collection.json
will be downloaded. Save it to the newly created directory.
You also need to export the Postman environment you created for this tutorial. Click the environment tab from the sidebar and make sure that the my-remote-api-testing
is selected. Click the menu icon and export your environment from the pop-up dialog.
A file named my-remote-api-testing.postman_environment.json
will be downloaded. Save this file to the newly created directory as well.
Note: If you use different filenames, be sure to stay consistent, or rename the files using the examples in the tutorial.
Automating the testing process using the Newman orb
The final step in this tutorial is to write the test automation script. This script will use Postman’s CLI sibling, Newman, to run the collection using the exported environment. Luckily, CircleCI has an orb for Newman. Orbs are packages that abstract a lot of boilerplate configuration. Orbs provide developers with a friendly API for invoking common commands and for using tools like Newman, Heroku, and environments.
Because newman
is a third-party orb, your CircleCI account must be set up to allow it. To change this setting, go to the Organization Settings of your CircleCI account. From the left sidebar, click Security. On the Security Setting page, select Yes to allow third-party orbs in your build.
Creating a configuration file
Create a folder named .circleci
at the root of the project and add a configuration file named config.yml
inside the folder. In this file, enter this code:
version: 2.1
orbs:
newman: postman/newman@1.0.0
jobs:
build:
executor: newman/postman-newman-docker
steps:
- checkout
- newman/newman-run:
collection: ./Users.postman_collection.json
environment: ./my-remote-api-testing.postman_environment.json
workflows: null
By calling the newman/newman-run
command, the script above loads Postman’s Newman
orb and runs the collection
using the specified environment
. It is just that simple.
Initialize a git repository, then commit your changes and push your project to GitHub.
Connecting the project to CircleCI
Now, log into your CircleCI account. If you signed up with your GitHub or GitLab account, it will be easy to connect your project.
On the Organization homepage, click Create Project. Select your project from the dropdown, give it a meaningful name, and click Create Project.
CircleCI will automatically detect your configuration file, but won’t run the pipeline. To trigger a pipeline run, push a small, meaningless commit. This will trigger the pipeline build, which runs successfully.
To review the test results, click the build and expand the newman run
tab.
As expected, Newman runs the collection, then generates a report detailing how the tests ran.
Awesome!
Conclusion
Manual testing process can quickly become a cumbersome, error-prone routine. The Postman GUI is already a must-have for every API developer’s toolkit. CircleCI automated pipelines and the newman
orb can take your API testing even further.
Happy coding!