Deploy Django apps to AWS Elastic Beanstalk
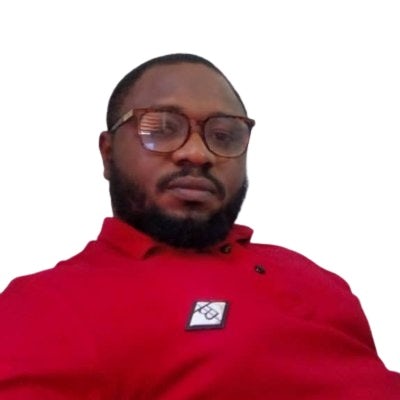
Fullstack Developer and Tech Author
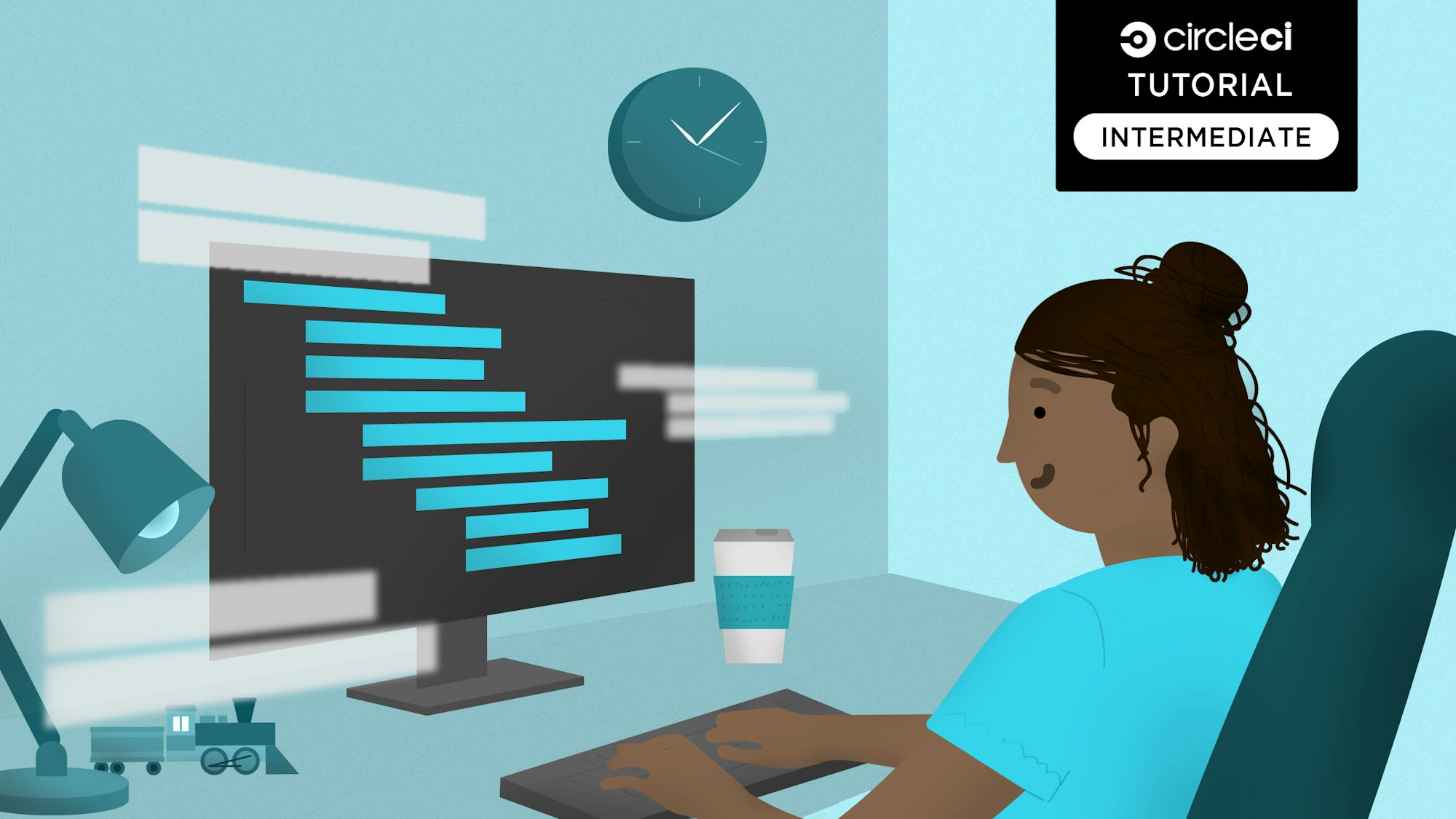
Your development team has access to countless tools, and as established tools are used in new ways, more options continue to emerge. For example, JavaScript has evolved from a simple web scripting language to a full-stack solution powering both frontend and backend development. Likewise, Python has gained traction in web development through frameworks like Flask and Django.
Django is especially popular for its “batteries included” feature set. These features – authentication, ORM, and admin interfaces – help developers build robust web applications quickly and efficiently.
In this article, you will learn how to deploy a Django application to AWS Elastic Beanstalk, a fully managed service that makes deploying and scaling web apps fast and painless. To further simplify the process, you’ll build a CI/CD pipeline using the CircleCI AWS Elastic Beanstalk orb to automate both authentication and deployment.
Prerequisites
In addition to a basic understanding of Django and Python, you will need these items:
- A GitHub account account
- A CircleCI account
- An AWS account
- The AWS Elastic Beanstalk CLI installed on your computer
Cloning the demo project
Clone the sample Django project by running this command:
Next, go to the root of the new folder you just created. Set up the project and run the local sever with these commands:
By default, your application will be served to http://127.0.0.1:8000/
. You can review the JSON response at that endpoint.
Reviewing the deployment strategy
Now that you have the application running locally, it is a good idea to review your deployment strategy. There is no need for any project code changes other than including the configuration file to set up deployment for CircleCI.
Here is a list of the deployment strategy steps:
- Deploy the app to Elastic Beanstalk
- Create a CI/CD configuration file to build the application on CircleCI
- Push the project to a repository on GitHub
- Add Amazon credentials to your pipeline to authenticate using the AWS Elastic Beanstalk orb
- Connect your project repository to your CI/CD pipeline and trigger a new deployment
Deploying the app to Elastic Beanstalk
In the project directory, create a new directory named .ebextensions
.
In the .ebextensions
directory, create a new file named django.config
and add this to it:
The WSGIPath starts with the directory containing your wsgi.py script. It will be used to start your application.
Create an AWS user with programmatic access
To configure AWS EB CLI you need an AWS user with programmatic access. Skip to the next step if you already have an AWS IAM user with access keys. To add a programmatic user, go to the AWS Add User page. Add a user name and click next.
On the permissions page, select “Attach Policies Directly” and then select “AdministratorAccess”” and click next.
On the “review and create” page, ensure you have a view similar to the one below and then click “create user”.
You’ll be taken to a page with all AWS users for your account. Click the new user to grant it programmatic access.
Next click the Security credentials tab, then the Create access key button.
Select Command Line Interface (CLI) from the list of options.
Review the newly-generated access key. Click the Download.csv to download the credentials in a CSV file format.
Configure the Elastic Beanstalk CLI
Next, initialize your EB CLI repository using this command:
If you do not have the credentials already configured, you will be prompted to provide the aws-access-id
and aws-secret-key
. Enter the values contained in the downloaded CSV file from the previous step. This creates a .elasticbeanstalk
folder with a config.yml
file containing AWS EB configurations.
Create a new AWS Elastic Beanstalk environment
Create a new environment using this command:
This creates an Elastic Beanstalk environment named django-env
; it takes about five minutes. The deploy will likely fail, which is expected at this point.
Once the process is completed, you can find the domain for the new environment by running this command:
The domain name is the value of the CNAME
property.
Once the application has been deployed, add the CNAME
property to the ALLOWED_HOSTS
setting in exchangeRateApi/settings.py
.
Because you are using Git for version control, you will need to commit your changes before running eb deploy
. Deploy the changes:
Now, run the eb status
command again. This time, the health status should be GREEN. Paste the CNAME
property in your browser to review your API.
Configuring CI/CD for automated deployment
Next, you’ll set up a CI/CD pipeline to automate your deployment process. This pipeline will allow you to push changes to AWS Elastic Beanstalk automatically after successful tests, saving time and removing manual steps from your workflow.
At the root of the project, create a new folder named .circleci
. In this folder, create a new file named config.yml
and add this:
version: 2.1
orbs:
python: circleci/python@3.0.0
eb: circleci/aws-elastic-beanstalk@2.0.1
jobs:
build:
description: "Setup Django application and run tests"
executor: python/default
steps:
- checkout
- python/install-packages:
pkg-manager: pip
- run:
name: "Run tests"
command: python manage.py test
workflows:
build-and-deploy:
jobs:
- build
- eb/deploy:
context: aws-credentials
application-name: exchangeRatesApi
environment-name: django-env
platform-version: python-3.9
requires:
- build
This configuration uses two orbs provided by CircleCI. The python
orb gives access to a Python environment (with pip
installed), which is used to test the updates before deploying them to AWS. Testing your software is an important prerequisite to automated deployments, as it ensures your code is stable and free of errors before going live, reducing the risk of failed deployments.
All of this happens in the build
job. The eb
orb deploys the changes to the environment you created in an earlier step.
After the workflow finishes running the build
job, the deploy
job from the eb
orb deploys the changes. The requires
key ensures that the build
job runs first. The application name, environment name, and platform version are specified for the job. A secure context named aws-credentials
is passed to the job. You will create this context later in the tutorial.
Commit your changes:
Finally, push your changes to your own remote repository on GitHub.
Adding AWS credentials to your pipeline
For your pipeline to run properly, you need to provide your AWS credentials to CircleCI so it can authenticate and deploy to AWS Elastic Beanstalk. This is done by securely storing your credentials in a context.
From your CircleCI dashboard, go to the Organization Settings page. Select Contexts, then click the Create Context button. Enter a unique name for your context. Your context appears in a list with security set to All members
. That means that anyone in your organization can access this context at runtime. As specified in the .circleci/config.yml
configuration for this tutorial, the context name should be aws-credentials
.
Next, select the aws-credentials
context.
Click the Add Environment Variable button and enter the variable name and value. Then click the Add Variable button to save. The aws-credentials
context requires 3 environment variables:
AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY
AWS_DEFAULT_REGION
The value of AWS_DEFAULT_REGION
is specified in the .elasticbeanstalk/config.yml
file in the project, under the global
section.
Connecting the application to CircleCI
Next, you need to set up a repository on GitHub and link the project to CircleCI. Review Pushing a project to GitHub for instructions.
Log into your CircleCI account. If you signed up with your GitHub account, all your repositories will be available on your project’s dashboard.
You will be prompted to enter the branch housing your configuration file.Enter the name of the GitHub branch and Click Set Up Project.
If your first pipeline doesn’t run automatically, commit and push a small change to trigger the first run.
To confirm that your workflow was successful, you can open your newly deployed app in your browser using the CNAME
property.
Conclusion
In this tutorial, you learned how to set up a CI/CD pipeline for a Django API using CircleCI and AWS Elastic Beanstalk. Using what you have learned here, you can implement fully automated deployments for your Python projects, saving you significant time and effort over the lifespan of your application. If your team has struggled to deploy projects that use their programming language of choice, this tutorial can open new opportunities.
The entire codebase for this tutorial is available on GitHub.