DevOps 101
What is a CI/CD pipeline?
8 min read
What is platform engineering? A quick introduction
8 min read
6 optimization tips for your CI configuration
11 min read
CircleCI News
Engineering Productivity
Most popular
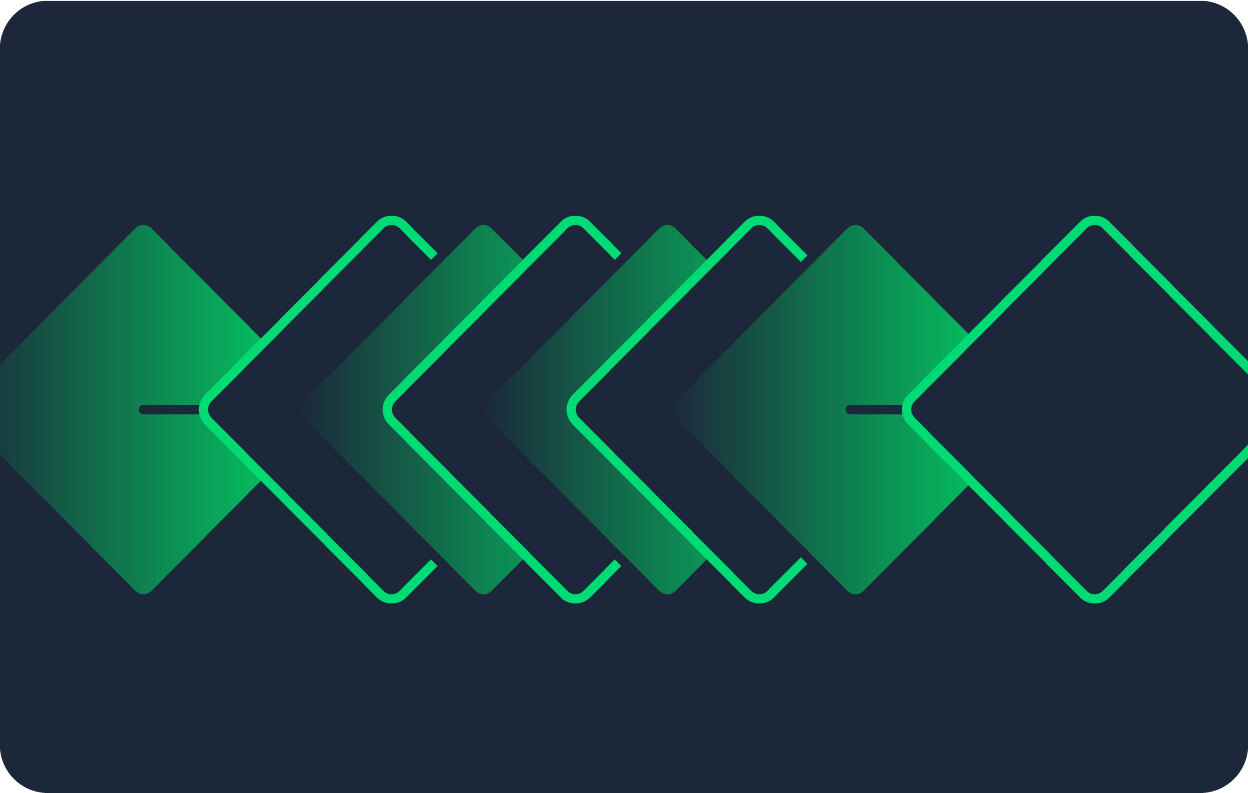
Build a scalable internal developer portal with Backstage and CircleCI
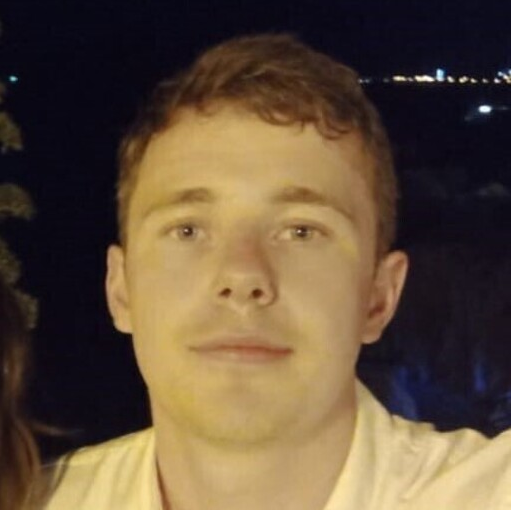
Senior Solutions Engineer
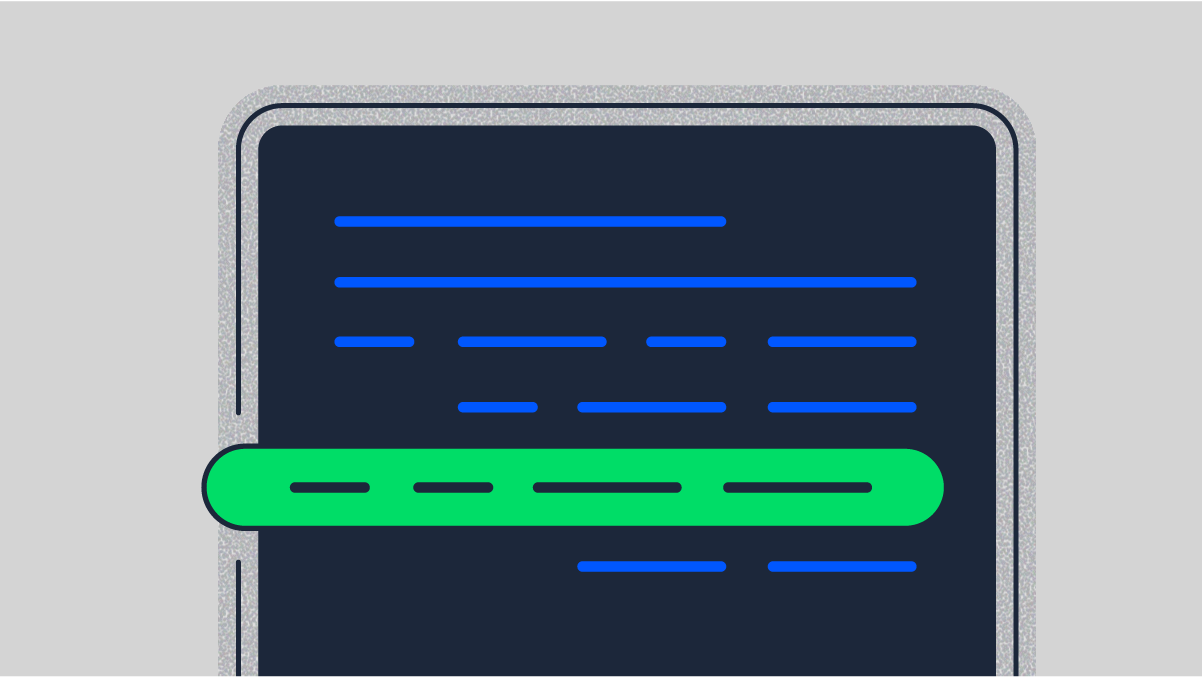
CircleCI MCP server: Natural language CI for AI-driven workflows

Principal Engineer
CI/CD at scale: A performance analysis of CircleCI vs GitHub Actions

Senior Field Engineer
Introduction to developer experience: What, why, and how

Senior Technical Content Marketing Manager