Deploying a React application to Netlify
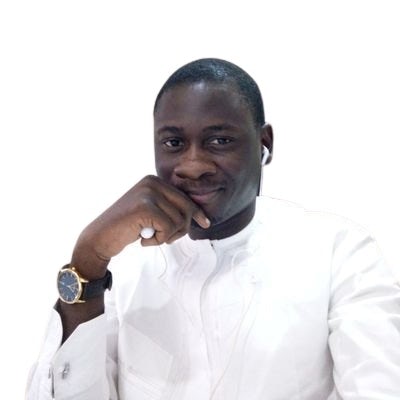
Fullstack Developer and Tech Author
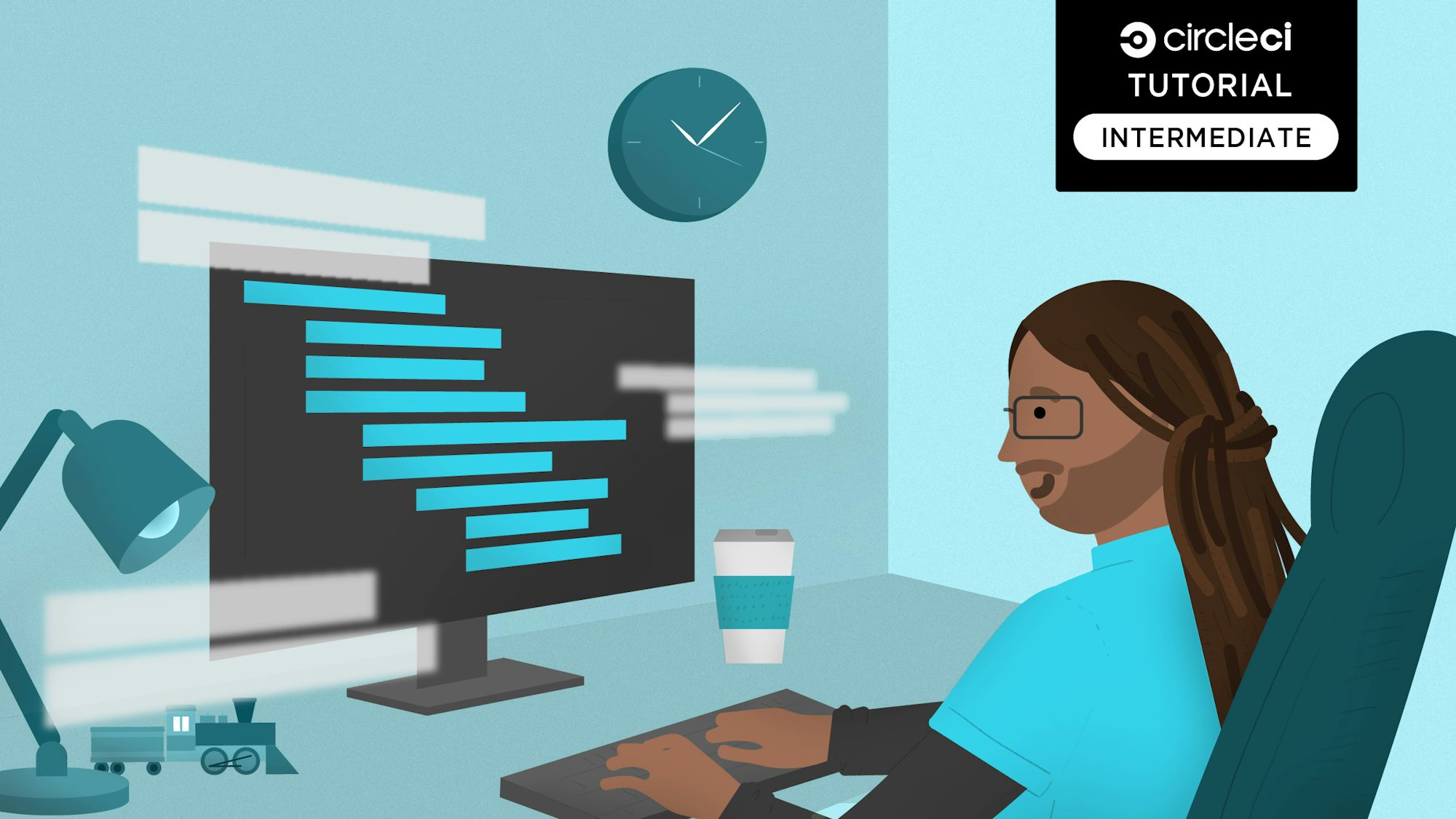
React is a front-end framework for building user interfaces that uses component-based architecture and non-opinionated design principles, making it a developer favorite. React has been widely adopted and has a large community of developers behind it. Netlify is a popular framework for hosting React applications, but it does not provide the highest level of control over the deployment process. As a result, your team may not be able to perform important tasks like running automated tests.
In this tutorial, you will learn how to take control over the deployment process by using CircleCI as your continuous deployment server. With the system you set up using this tutorial, you can perform any custom step you need to complete your build process and deploy your React application to Netlify.
Prerequisites
To follow along with this tutorial, you will need:
- Node.js installed on your system (version 18 or newer)
- A Netlify account
- A CircleCI account
- A GitHub account
Our tutorials are platform-agnostic, but use CircleCI as an example. If you don’t have a CircleCI account, sign up for a free one here.
With all these installed and set up, we can begin the tutorial.
Creating a new React site
Choose a location within your system to create the React site that will be deployed to Netlify. Run this command:
npx create-react-app cd-netlify-react-app
This command will invoke the create-react-app
npm package to scaffold a new React application.
The result is a React project created in the cd-netlify-react-app
folder. Use these commands to run the project:
cd cd-netlify-react-app
npm start
The commands boot up the React site at the local URL: http://localhost:8000
. Load this address in your browser.
Setting up the GitHub project
You need to set up your React project on GitHub before you can get it on Netlify and CircleCI. First, install the Netlify CLI package as a development dependency in your React project. Run this command at the root of your project:
npm install --save-dev netlify-cli
Note: Adding the Netlify CLI as a dependency prevents breaking changes for deployments in CI environments.
You can now push your project to GitHub. Once your project is on GitHub, create a new branch. Give this branch any name you want; for this tutorial I will name it netlify-deploy-ignore
.
You may be wondering why you need this branch. Netlify needs a project branch to automatically trigger an application’s deployment. When changes are pushed to this branch, Netlify will initiate the deployment process. You want to avoid a situation where both Netlify and your CircleCI pipeline are running two deployment processes in parallel.
This new branch acts as a decoy for Netlify to monitor. Do not push changes to this branch. Its purpose is to “distract” the Netlify deployment process so that you can use CircleCI for deployment. You can make this a protected branch on GitHub so that no one on your team pushes to it by mistake.
Creating the Netlify application
To create a new Netlify application, go to your Netlify dashboard){: target=”_blank”} and click the New Site from Git button. Next, select GitHub as your provider and search for the project.
Select the project and go to the next step to select a branch for Netlify to deploy from. Select your decoy branch.
Next, click the Deploy cd-netlify-react-app button so that Netlify performs the very first deployment of your site. Netlify will generate a random name for the deployed application. Click the link as shown on the dashboard. For example, mine is https://admirable-sfogliatella-070d98.netlify.app
There are two configuration values you will need to get from your Netlify app and account to perform automated deployments in your CircleCI pipeline.
- The
SITE ID
for the application you just created can be found in the Site Details section of your Netlify app. - The Personal Access token allows access to your Netlify account from your deployment pipeline. Generate an access token at
User Settings > Applications
.
Save your access token in a secure place because Netlify will not allow you another view of this value after you create. You will only be able to change it.
Automating the deployment
Time to set up your project on CircleCI. Add the .circleci
folder to the root of your project. In it, add a new config.yml
file and use the following content for it:
version: 2.1
jobs:
build:
working_directory: ~/repo
docker:
- image: cimg/node:18.16.0
steps:
- checkout
- restore_cache:
key: dependency-cache-{{ checksum "package-lock.json" }}
- run:
name: Install Dependencies
command: npm install
- save_cache:
key: dependency-cache-{{ checksum "package-lock.json" }}
paths:
- ./node_modules
- run:
name: Build React App
command: npm run build
- save_cache:
key: app-build-cache-{{ .Branch }}
paths:
- ./build
- run:
name: Deploy to Netlify
command: ./node_modules/.bin/netlify deploy --site $NETLIFY_SITE_ID --auth $NETLIFY_ACCESS_TOKEN --prod --dir=build
workflows:
version: 2
build-deploy:
jobs:
- build:
filters:
branches:
only:
- main
In this configuration, the project is checked out of the repository and the project dependencies are installed and cached. After caching the dependencies, the React build command npm run build
runs. It creates a production build of the application in the build
directory at the root of the project, which is then cached.
Finally, the Netlify CLI deploys the site using the $NETLIFY_SITE_ID
and $NETLIFY_ACCESS_TOKEN
variables. The workflow configuration then ensures that only the main
branch triggers a deployment to Netlify.
Before you push this configuration for CircleCI to deploy the site, go Change the React message in src/App.js
file within the <p>
tag to this:
<p>Successfully Deployed <code>A React application</code> to Netlify with CircleCI</p>
This enables you to view a change in the deployed application.
Finally, commit all your changes and push to GitHub.
Setting up the project on CircleCI
Log into your CircleCI account. If you signed up with your GitHub account, all your repositories will be available on your project’s dashboard. Search for the cd-netlify-react-app
project:
Click Set Up Project. You will be prompted about whether you have already defined the configuration file for CircleCI within your project. Enter the branch name (for the tutorial, you are using main). Click Set Up Project to trigger your build pipeline for the first time.
The build will fail because you have not added the Netlify credentials.
To fix that, click the Project Settings button. On the settings page, select Environment Variables from the side menu. On this new page, click the Add Environment Variable button and enter this information:
NETLIFY_SITE_ID
: The API ID from your Netlify applicationNETLIFY_ACCESS_TOKEN
: Your Netlify personal access token.
Click Rerun Workflow from Start.
This will again trigger the deployment pipeline and a successful build.
Click the build to review the deployment details.
With this successful build, visit your website again to verify the changes you deployed. It should display the new message.
Awesome!
To confirm that Netlify is not running a parallel deployment, check your Netlify deployment logs. There is only one deployment in the Production deploys, the first one that was triggered by the branch netlify-deploy-ignore
. The rest don’t show a branch because they were triggered by the CircleCI pipeline.
Conclusion
There you have it, custom deployment of a React site to Netlify using CircleCI. This setup gives you more flexibility and control of your React deployments to Netlify. That gives your team the ability to run more custom steps within the process. The complete source code can be found here on GitHub.
Happy Coding!