Automatically deploy Svelte applications to Heroku
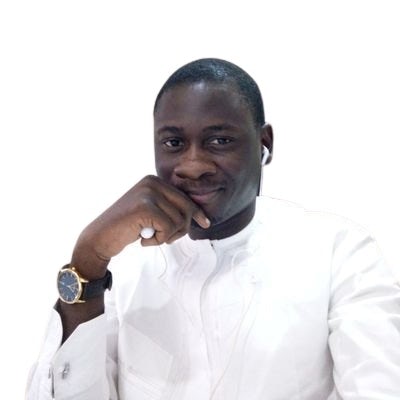
Fullstack Developer and Tech Author
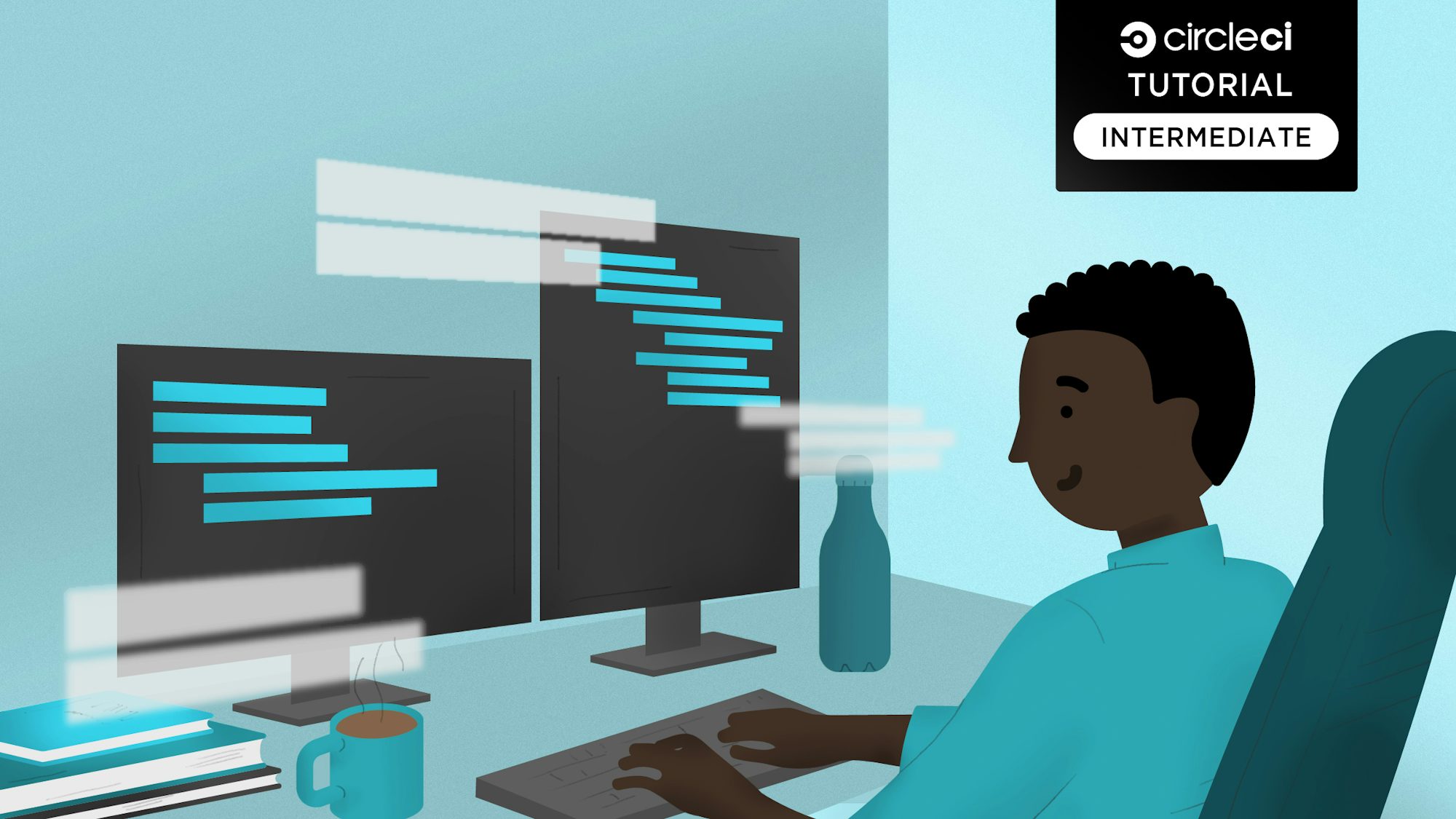
While React, Vue, and Angular take center stage among JavaScript front-end frameworks, Svelte disrupts the equilibrium of the space by solving problems in a unique way. Unlike other frameworks, Svelte does not do its DOM-updating work in the browser using the Virtual DOM. Instead, it compiles efficient JavaScript code in its build step that efficiently updates your DOM when a state change occurs.
In this tutorial, we will create an automated continuous deployment (CD) pipeline that deploys our Svelte app to Heroku. You can pair this tutorial with Continuous integration for Svelte applications to build a complete continuous integration and continuous deployment (CI/CD) solution for your Svelte development workflows.
Prerequisites
To follow along with this tutorial, a few things are required:
- Basic knowledge of JavaScript
- Node.js installed on your system
- A Heroku account
- A CircleCI account
- A GitHub account
Our tutorials are platform-agnostic, but use CircleCI as an example. If you don’t have a CircleCI account, sign up for a free one here.
With all these installed and set up, let’s begin the tutorial.
Creating a Svelte project
Choose a location within your system. To create a new project there, run:
npm create svelte@latest svelte-sample-heroku-app
This command uses SvelteKit to scaffold a new Svelte project. This is the approach recommended by the Svelte team.
You will prompted to provide details of the app. Respond this way:
create-svelte version 5.1.1
┌ Welcome to SvelteKit!
│
◇ Which Svelte app template?
│ SvelteKit demo app
│
◇ Add type checking with TypeScript?
│ Yes, using TypeScript syntax
│
◇ Select additional options (use arrow keys/space bar)
│ Add ESLint for code linting, Add Prettier for code formatting, Add Playwright for browser testing, Add Vitest for unit testing
│
└ Your project is ready!
✔ Typescript
Inside Svelte components, use <script lang="ts">
✔ ESLint
https://github.com/sveltejs/eslint-plugin-svelte
✔ Prettier
https://prettier.io/docs/en/options.html
https://github.com/sveltejs/prettier-plugin-svelte#options
✔ Playwright
https://playwright.dev
✔ Vitest
https://vitest.dev
This command will begin scaffolding a new Svelte project. Once the scaffolding process is done, go into the root of your project (cd svelte-sample-heroku-app
) and run npm install
to install the required packages. Once the installation is complete, you will need to boot up a server to serve the application in development mode. Run:
npm run dev
This serves the application at the http://localhost:5173/
address.
Note: 5173
is the default port. If it is in use, a different port will be assigned.
You now have a functional Svelte app up and running.
Setting up Heroku for deploying Svelte apps
To set up a Heroku application, sign into your account and create a new application.
You will need the name of your application and the API key for your account. The name will svelte-sample-heroku-app
, or the name you used when you created the application. You can find the API key in the Heroku Account Settings section. You will use these two values (the app name and API key) later on to create environment variables on CircleCI for deployment to Heroku.
While you have your Heroku account open, install the buildpack for the project. Buildpacks are scripts that run when your application is deployed. You will need the Node.js buildpack for this tutorial.
heroku/nodejs
: Required for all Node.js applications
Click the Settings tab. In the Buildpacks section, click Add buildpack.
A form allows you to select an officially supported buildpack or provide a URL for one. Select nodejs to use the official Heroku Node.js buildpack. Click Save changes. Node.js will be used to build your next deployment.
Go to your Heroku application Settings page and scroll down to the Buildpacks section (you might find that heroku/nodejs
buildpack has already been added). Click Add Buildpack.
For each buildpack (heroku/nodejs
and heroku/heroku-buildpack-static
) enter the identifier/URL into the text field and click Save changes.
Note: You can skip this step if the heroku/nodejs
buildpack has already been added.
That completes the set up required on Heroku.
Installing the SvelteKit app adapter
Next, you need to adapt your application for the deployment environment. In this part of the tutorial, you will generate a standalone Node server for the Heroku environment.
For the SvelteKit app, Adapters are plugins that take the built app as input and generate deployment output.
To install an adapter for Node servers, run:
npm i -D @sveltejs/adapter-node@next
Once the installation is done, modify the svelte.config.js
file to use the Node adapter:
import adapter from "@sveltejs/adapter-node";
import { vitePreprocess } from "@sveltejs/kit/vite";
/** @type {import('@sveltejs/kit').Config} */
const config = {
preprocess: vitePreprocess(),
kit: {
adapter: adapter(),
},
};
export default config;
Open package.json
file and add a start script to run the application on Heroku once its deployed:
...
"scripts": {
"start": "node build/index.js"
},
...
Automate deployment of the Svelte app using CircleCI
In this section, you will create the configuration file to deploy your application to Heroku using CircleCI.
The most important configuration parameter here is the root
property. A Svelte application is served via a public
folder. Therefore, the root
property is used to point to this folder so that the host serves the correct code. https_only
is specified to ensure that our application is always served via a secure protocol.
Now you can write your deployment script. At the root of your Svelte project, create a folder named .circleci
and a file within it add a file named config.yml
. Inside the config.yml
file, enter this code:
version: "2.1"
orbs:
node: circleci/node@5.1.0
jobs:
build-and-deploy:
docker:
- image: "cimg/base:stable"
steps:
- checkout
- node/install
- run:
command: npm install
name: Install dependencies
- run:
name: Deploy app to Heroku
command: |
git push https://heroku:$HEROKU_API_KEY@git.heroku.com/$HEROKU_APP_NAME.git main
workflows:
deploy:
jobs:
- build-and-deploy
This configuration uses Git to deploy the latest code changes to your Heroku account.
Next, get your project set up on CircleCI. Begin by pushing your project to GitHub.
Log into your CircleCI account. If you signed up with your GitHub account, all your repositories will be available on your project’s dashboard.
Click Set Up Project next to your svelte-sample-heroku-app
project.
You will be prompted to enter the name of the branch where your configuration file is.
Click Set Up Project once you are done to trigger your first workflow. This workflow will fail because you have not provided your Heroku API key yet.
Provide the Heroku API now. Click the Project Settings button, then click the Environment Variables menu option. Add two new variables.
-
For
HEROKU_APP_NAME
add the app name you used in Heroku. The name will be eithersvelte-sample-heroku-app
or a custom name, if you created one. -
For
HEROKU_API_KEY
enter the Heroku API key that you retrieved earlier from the account settings page.
Re-run your workflow from the start, and this time your workflow will run successfully.
To confirm that your workflow was successful, open the newly deployed app in a browser. The URL for your application should be in this format https://<HEROKU_APP_NAME>-<RANDOM_NUMBER>.herokuapp.com/
. You can find the generated domain name for your app on the Settings page.
Awesome!
Your Svelte application is live on Heroku.
Conclusion
You have deployed a Svelte application to Heroku using CircleCI to build an automated continuous deployment pipeline. To deploy updates to your application, you need only push your code and all changes will deploy automatically. This exercise also demonstrates how CircleCI makes setting up deployment easy.
The complete source code for the project built in this tutorial can be found here on GitHub.
Happy coding!