Continuous integration for mobile application development

Senior Manager, Marketing Insights and Strategy
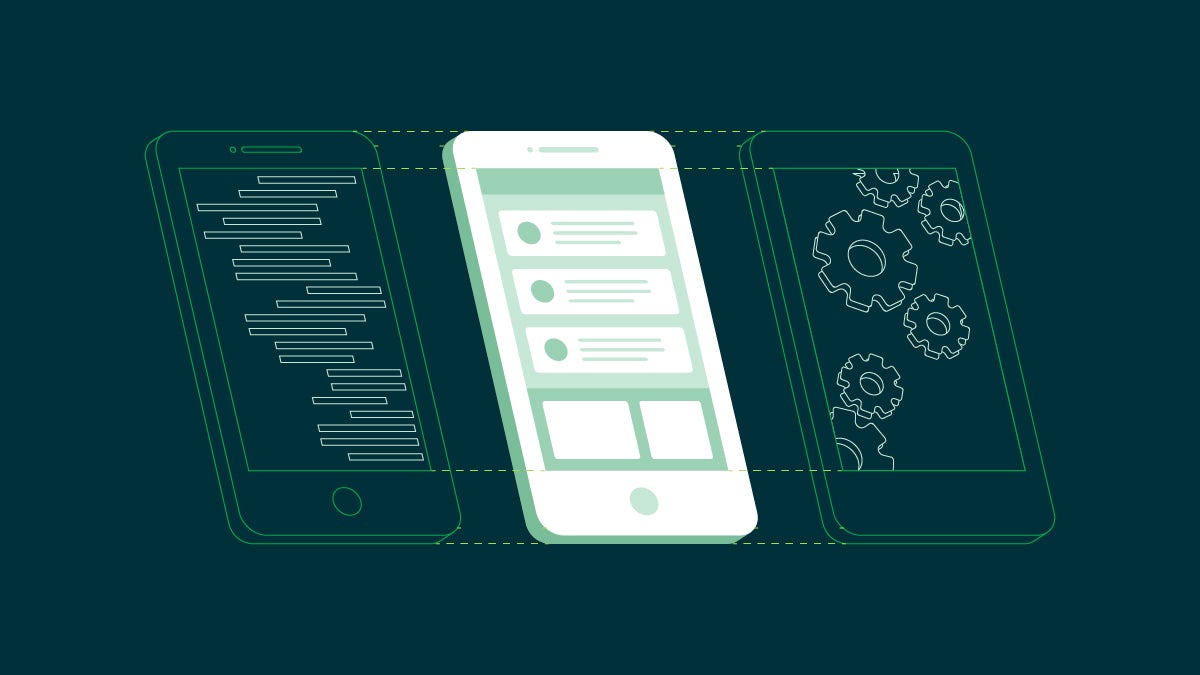
Testing is a critical part of software development. Testing mobile applications can be more difficult than testing server or web-based applications, because to check the app’s functionality, the app must run on a physical Android or iOS device or in a simulator.
Fortunately, by automating your mobile application tests using continuous integration (CI) tools, the process can become much easier, more efficient, and more consistent.
Let’s go through an overview of how we can set up a mobile application for testing and explore how to automate the building and testing process with a CI pipeline.
How to create a mobile app with tests
Creating a testing-ready mobile application is supported by both Android Studio and Xcode (iOS) with all new projects. Each IDE provides a built-in way to add and run tests — more specifically, to add unit tests.
For Android applications, Android Studio creates a directory (/src/test/java/) with a new project where we can write tests as an implementation of the JUnit 4 test class. We also need to configure several dependencies in the build.gradle
file. You can refer to this Google guide for more detailed instructions on how to create an Android app with tests.
For iOS applications, Xcode includes a test target in the project scheme with stub methods for a unit test when you create a new project. We can write tests inside this target and then run them through the menu by choosing Product > Test, or select and run a subset of them by choosing Product > Perform Action > Run Test Methods. Take a look at Apple’s guide on Using Unit Tests and About Testing with Xcode for more information.
A mobile app CI solution will be able to build these projects and run its tests with a simple configuration.
Testing and automating a mobile application
Looking at types of tests, testing a mobile app happens at several segregated testing environments:
- Unit Tests - Small-scale tests for parts of our code, which do not need to run on a device and can run on the build machine.
- Integration Tests - Higher-level tests that could interact with native device functionality such as geolocation and need to run on a device or simulator.
- Functional and UI Tests - Tests to ensure the app runs and functions properly and must be run on a device or simulator, such as scene navigation.
- Performance Tests - Stress tests to measure app performance metrics.
As part of configuring a CI pipeline, we can automate the process of building the code and running these tests on each new code committed to the repository. CI typically runs as a server on the cloud or locally on your machine, and it builds and runs the configured commands or tasks.
Working with unit tests, we can use Android Studio with JUnit or Xcode with XCTest or, if we prefer, other standard Java and ObjC/Swift test frameworks, because they can run directly on the build machine.
For other tests that need to run on-device or on a simulator, tools like Appium can easily create app tests such as UI automation.
By integrating these tests with CircleCI, for example, we can integrate and automate our build and test process by adding the project repository to the CircleCI application and setting up a config.yml
file to define steps that will pull the latest code from the repository, build it, and then deploy and run the mobile application and/or its tests.
An example configuration file looks like this:
# .circleci/config.yml
version: 2.1
jobs:
build-and-test:
macos:
xcode: 11.3.0
environment:
FL_OUTPUT_DIR: output
FASTLANE_LANE: test
steps:
- checkout
- run: bundle install
- run:
name: Fastlane
command: bundle exec fastlane $FASTLANE_LANE
- store_artifacts:
path: output
- store_test_results:
path: output/scan
We encourage using Fastlane with CircleCI because it simplifies the setup and automation of the build, test, and deploy process, and works with both iOS applications and Android applications. Read Building and deploying Flutter apps with Fastlane for more details on using Fastalne with CIrcleCI.
And by using CircleCI orbs, it is especially easy to get started with mobile test automation. Rather than spending significant amounts of time trying to figure out how to integrate with different services and specifying jobs and commands, we can add orbs, which are shareable packages of configuration elements, to use directly in our CI pipeline. The orbs catalog gives us plenty to choose from.
Mobile app security concerns
CI can help alleviate critical security concerns in addition to simplifying testing as part of the mobile development process.
Take a look at these examples where CI could address potential security issues:
- Credentials and Authentication - In a team or organization, keeping account passwords and permissions safely restricted to administrators can be important. Running sensitive commands or tasks such as code signing with a private certificate or uploading a build using an API key will be limited to the CI server.
- Data Encryption - Personal user information and passwords need to be handled with care, so tests making sure this data is always encrypted in the device and over the web will help protect our users through code changes.
- Feature Regression - One of the best ways to keep former bugs and issues fixed and not reintroduce them into our code is to create an automated test that checks for the scenario with each build.
- Error Handling - Mobile app crashes and memory buffer limits can be a source of security exploits or at least a bad user experience. Tests for graceful error handling can mitigate these issues.
- Device Features and Permissions - Tests can help ensure that our app uses native device functionality such as the microphone or camera properly and only during the correct app scenes when the user intends to be recorded, never outside of that context. It could be a similar case with the app gaining permission access to the user’s saved photos or files.
Deploying mobile apps via CI
Finally, once our mobile app has been tested and is ready for release, CI can also help by publishing it directly to app stores.
From a high-level view, a CI task could grab the latest version of the code, increment the version number, build the project source for release, and then upload the packaged app directly to the Google Play Store or the Apple App Store.
We recommend using Git branches for this scenario and specify a release branch so that we can keep it separate from a development branch and allow CI to upload builds to the stores only once code is merged from the development branch to the release branch.
Mobile application deployment is easily configured using CircleCI together with Fastlane. For iOS applications, we can provide access to App Store Connect and a Fastlane configuration with steps to build and upload the release version of the app. Fastlane also makes it possible to generate screenshots during the process for metadata using its screenshot and frameit actions.
Follow the steps in the CircleCI documentation to configure mobile app deployment on iOS and on Android.
Getting started with mobile development
We just walked through a high-level view of the steps involved in automating our mobile app tests using CI, and as we’ve seen, this does not have to be difficult. It makes sense to start mobile app projects the right way: with testing and CI built in from the beginning.
With CircleCI, automated app testing and deployment can be easy. Even better, it does not cost anything to get started, and you can apply for a special plan with free monthly build credits if your project is open source.
Before we wrap up, here are some useful resources that could help you begin your automated mobile app testing journey with CircleCI:
- Mobile and browser testing on CircleCI: simple setup, easy management, increased confidence
- Mobile integrations with orbs
- Hello World examples
- Sample
config.yml
files - Continuous integration for iOS and Android apps
- iOS continuous integration: getting started
Good luck testing and automating your mobile application!